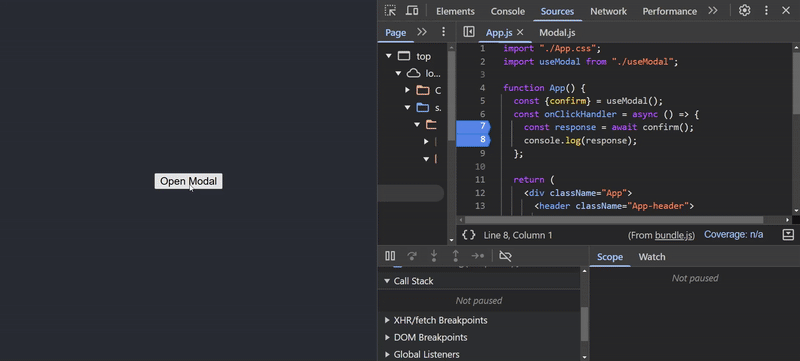
A Bottom-up Approach to Async Modals in React
In React, you can use combination of Context and custom hooks to implement async modals. Async modals let you capture the response from modal asynchronously, saving the hassle of managing another state and improves readibility. Let’s try to build it from the ground up: 1. Bare bones App.js function App() { const [isModalOpen, setIsModalOpen] = useState(false); const onResponseClick = (response) => { setIsModalOpen(false); console.log(response); }; return ( <div> {isModalOpen && <Modal onResponseClick={onResponseClick} />} <button onClick={() => { setIsModalOpen(true); }} > Open Modal </button> </div> ); } To get the response (yes/no) from the modal, App component is forced to manage isModalOpen state and include <Modal /> component in its JSX. We know there is a better way to manage this by using Context. ...